Organizing Files Automatically with Python: An Expanded Guide
Transform chaos into order: Discover how our expanded guide to automatically organizing files with Python can streamline your digital life and boost your productivity.
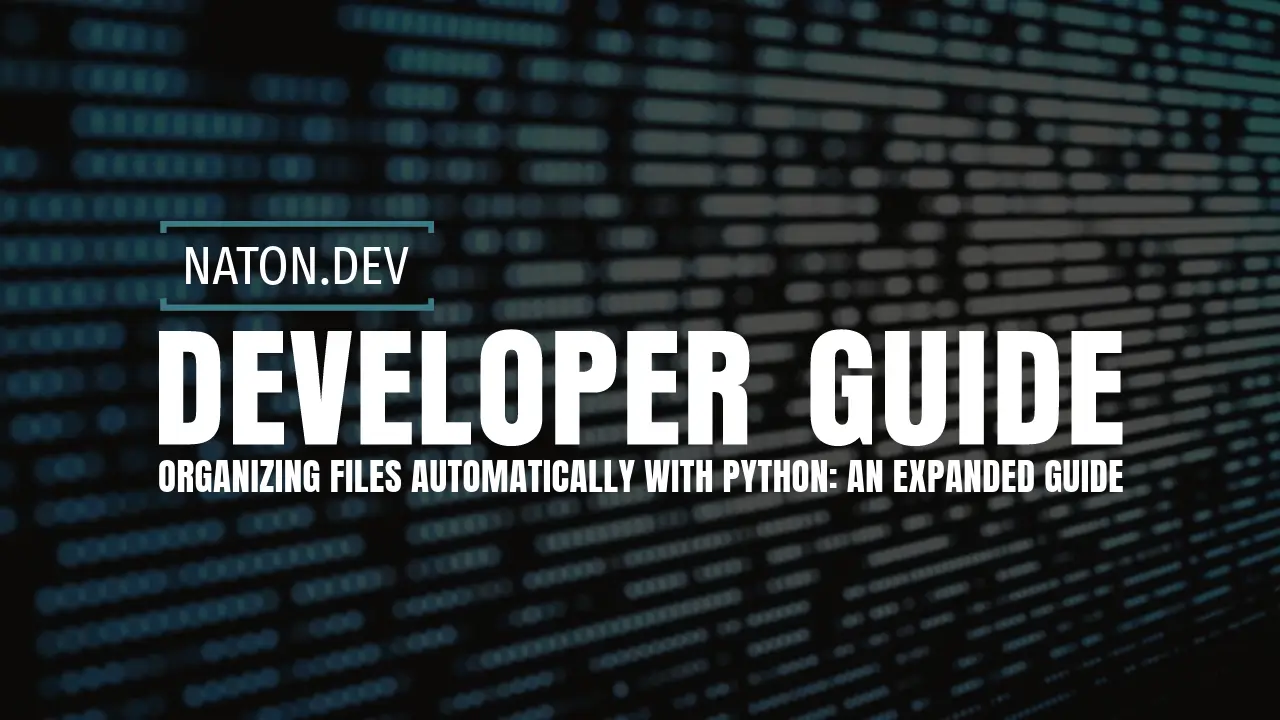
In today's fast-paced digital environment, managing the deluge of files we accumulate over time—be it downloads, documents, images, or miscellaneous files—can become an overwhelming task. These files, often haphazardly saved to our download folders or desktops, can create an environment of digital clutter that reduces efficiency and productivity. This guide introduces a Python-based solution to automate the organization of files by type, making it easier to maintain a tidy and functional digital workspace.
Objective
The aim here is to develop a Python script capable of sorting files within a chosen directory (e.g., your Downloads folder) into respective subfolders based on their file extension types. This automation will facilitate a more organized directory structure, making file retrieval straightforward and saving significant time otherwise spent on manual organization.
Getting Started
Prerequisites
Ensure Python is installed on your system. This script is built using Python's standard library, requiring no additional packages for basic file organization tasks.
Step-by-Step Guide
Step 1: Import Necessary Modules
To handle file and directory operations, we'll use two modules from Python's standard library: os
and shutil
. The os
module allows for interaction with the operating system to perform directory and file operations, while shutil
module provides high-level operations on files and collections of files.
import os
import shutil
Step 2: Define the Target Folder
Identify the specific folder you wish to organize. For the purpose of this guide, we will use the Downloads folder as an example. Ensure to replace '/path/to/your/downloads/folder'
with the actual path to the folder you wish to organize on your system.
folder_path = '/path/to/your/downloads/folder'
Step 3: Categorize Files by Extension
This crucial step involves iterating over each file within the target directory, categorizing them by their extension, and subsequently moving them to their designated subfolders.
def organize_files_by_extension(folder_path):
# Loop through each file in the directory
for filename in os.listdir(folder_path):
# Construct the absolute path of the file
file_path = os.path.join(folder_path, filename)
# Skip if it's a directory
if os.path.isdir(file_path):
continue
# Extract file extension and define the destination subfolder
file_extension = filename.split('.')[-1].lower()
destination_folder = os.path.join(folder_path, file_extension)
# Create the destination subfolder if it does not exist
if not os.path.exists(destination_folder):
os.makedirs(destination_folder)
# Relocate the file to its new subfolder
shutil.move(file_path, destination_folder)
print(f'Moved: {filename} to {destination_folder}/')
Step 4: Execute the Function
Now, run the function with the path to the directory you're organizing as its argument.
organize_files_by_extension(folder_path)
Additional Enhancements
- Handling Files Without Extensions: Implement logic to deal with files lacking extensions by assigning them to a 'Miscellaneous' or 'Other' folder.
- Customizable Organization Criteria: Extend the script to organize files based on additional attributes such as file size, creation or modification dates, or specific naming conventions.
- Detailed Logging: Incorporate logging functionality to create a record of file movements and to log errors. This can be invaluable for debugging and verifying the script's actions.
Usecases for Automated File Organization
The automated file organization tool developed using Python is not only a powerful asset for personal digital hygiene but also offers a range of applications in professional and academic environments. Below are several use cases that highlight the versatility and utility of this tool:
Personal Digital Cleanup
- Downloads Folder Management: The most direct application, it keeps your downloads folder meticulously organized, ensuring that files are easy to find and your digital workspace remains clutter-free.
- Photo Organization: Automatically sort a large collection of photos into subfolders based on file type or even date, if extended to read metadata.
Professional Workspace Enhancement
- Document Management: For professionals inundated with various types of documents, this tool can automatically categorize and store PDFs, Word documents, spreadsheets, and presentations into their respective folders, streamlining the retrieval process.
- Project File Sorting: Developers and designers can use the script to organize project files, such as code files, design assets, and documentation, ensuring a clean and efficient project structure.
Academic Material Organization
- Research Paper Sorting: Academics and students can manage their digital libraries by automatically sorting research papers, articles, and reference materials into subject-specific folders.
- Coursework and Lecture Notes: Keep lecture notes, assignments, and course materials organized by course name or year, making study materials more accessible and manageable.
Small Business Applications
- Invoice and Receipt Management: Automatically sort invoices and receipts into dedicated folders, aiding in financial organization and making tax preparation easier.
- Customer or Client File Organization: Sort client-related documents, contracts, and communication logs into individual client folders, improving customer service and operational efficiency.
Creative Works Archiving
- Art Portfolio Management: Artists can organize their digital portfolios by medium, project, or year, making it easier to archive and retrieve their work.
- Writing and Drafts Organization: Writers can automatically sort their drafts, research, and manuscripts by genre, project, or stage of completion.
Coding and Development
- Source Code Management: Organize source code files, libraries, and documentation by programming language or project, facilitating a more efficient development process.
- Resource Library Curation: Developers maintaining a collection of resources, such as tutorials, libraries, and tools, can keep these resources neatly organized.
Expanding the Tool
The script's flexibility allows for customization to fit specific organizational needs or integration into larger systems. For example, it could be adapted to monitor a folder continuously and organize files in real-time, or it could be integrated into a web application for server-side file management.
By automating mundane tasks like file organization, individuals and organizations can significantly improve productivity, reduce time wasted on searching for files, and maintain a high level of digital hygiene. This Python script is just a starting point, inviting further customization and adaptation to a wide array of use cases across different domains.
Conclusion
This Python script represents a starting point towards achieving a more organized and efficient digital workspace. By automating the task of file organization, you free up time to focus on more productive activities. Moreover, this guide lays the foundation for further exploration into Python's capabilities for file and directory management, encouraging the development of more complex and personalized automation scripts to suit a variety of organizational needs. With Python, the possibilities to enhance your digital organization are vast and limited only by your imagination.
Additional Learning Resources
Enhance your Python automation skills further with these handpicked resources:
- Python Official Documentation: Dive deep into Python's built-in modules for file and directory operations by exploring the official documentation. It's the best place to understand the core functionalities directly from the source.
- Automate the Boring Stuff with Python by Al Sweigart: This book is a treasure trove for anyone looking to use Python to automate mundane tasks, including file organization. Al Sweigart covers the essentials in a way that's accessible to beginners and valuable for experienced programmers.
- Real Python: Offers a wealth of tutorials and articles on Python for all skill levels. Their tutorials on file handling and automation are particularly useful for applying Python to everyday tasks.
These resources are a great starting point for enhancing your understanding and capabilities in Python automation. The official Python documentation will give you a solid foundation in file and directory handling, while "Automate the Boring Stuff with Python" provides practical applications and inspiration for automation projects. Real Python's tutorials offer in-depth guides and best practices to help you refine your skills further.
Remember, the journey of learning Python is continuous, and these resources are just the beginning. Explore, experiment, and expand your knowledge to develop more complex and efficient automation solutions.