How to Use ChatGPT with Python in 2023: A Beginner's Tutorial
Master the integration of ChatGPT with Python through this beginner-friendly tutorial, unlocking the potential of AI-driven text generation and automation in your projects.
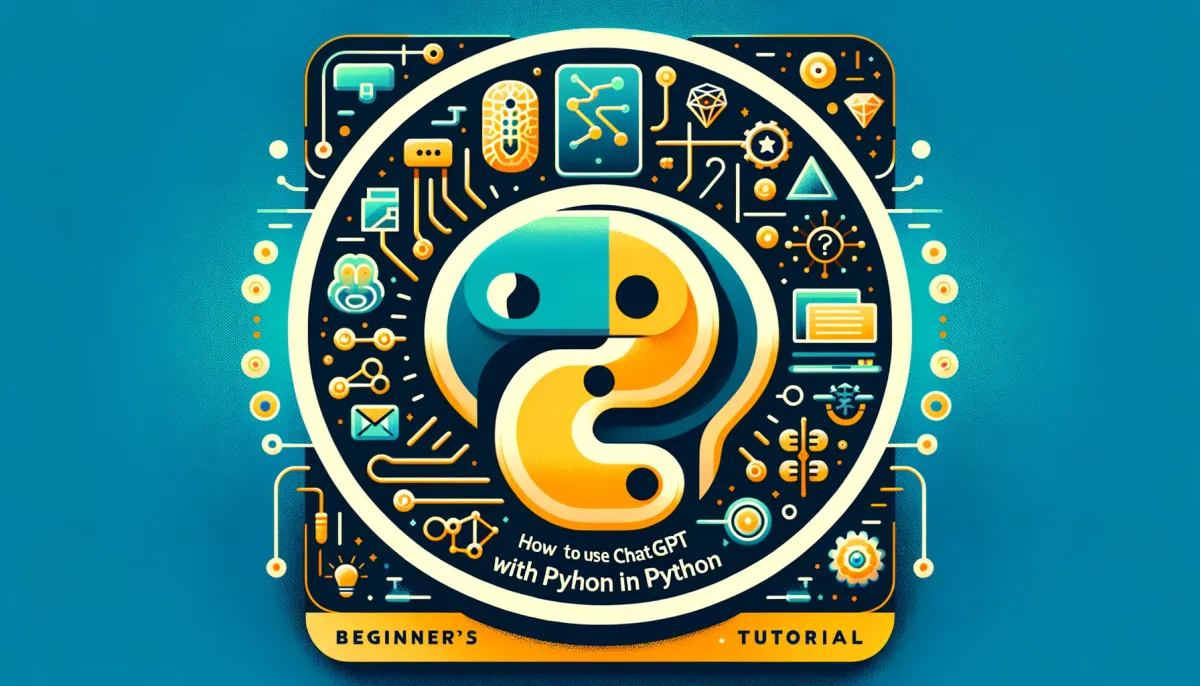
In the realm of artificial intelligence and natural language processing, ChatGPT by OpenAI has emerged as a groundbreaking technology. This tutorial is designed for beginners who wish to learn how to integrate and use ChatGPT within their Python projects in 2023. Whether you're looking to develop chatbots, automate customer service, or generate content, this guide will provide you with the foundational knowledge needed to get started.
Introduction to ChatGPT
ChatGPT is a variant of the GPT (Generative Pre-trained Transformer) models developed by OpenAI, designed specifically for understanding and generating human-like text based on the input it receives. It's capable of performing a wide range of language-based tasks, making it an invaluable tool for developers.
Setting Up Your Environment
Before diving into the code, ensure your Python environment is ready. You'll need Python installed on your system (Python 3.6 or newer is recommended). Additionally, you should have pip
available to install packages.
Step 1: Install OpenAI Python Package
First, you need to install the OpenAI Python package, which provides a convenient way to interact with the ChatGPT API. Open your terminal or command prompt and run the following command:
pip install openai
Step 2: Obtain API Key from OpenAI
To use ChatGPT, you'll need an API key from OpenAI. Visit the OpenAI website, sign up or log in, and navigate to the API section to get your API key. Keep this key secure, as it will be used to authenticate your requests to the API.
Integrating ChatGPT with Python
Once you have your environment set up and your API key ready, you're all set to start coding. The following sections will guide you through the process of sending a prompt to ChatGPT and receiving a response.
Step 1: Import OpenAI Package
Start by importing the OpenAI package into your Python script:
import openai
Step 2: Authenticate with Your API Key
Use your API key to authenticate your requests. For security reasons, it's best not to hard-code your API key into your scripts. Instead, use an environment variable or a configuration file. For this tutorial, we'll set it directly for simplicity:
openai.api_key = 'your_api_key_here'
Step 3: Sending a Prompt to ChatGPT
Now, let's send a prompt to ChatGPT and get a response. You can customize the prompt to fit your needs. Here's a simple example:
response = openai.Completion.create(
engine="text-davinci-003", # Or the latest available model
prompt="What is the capital of France?",
max_tokens=50
)
Step 4: Displaying the Response
Finally, print out the response from ChatGPT to see the result:
print(response.choices[0].text.strip())
Conclusion
Congratulations! You've successfully integrated ChatGPT into your Python project. With this basic setup, you can start exploring the vast capabilities of ChatGPT, from generating text to answering questions and much more. As you become more familiar with its functionalities, you can delve deeper into the API's documentation to discover advanced features and customization options. Happy coding!
Remember, the landscape of AI and machine learning is constantly evolving, so stay updated with the latest models and practices to ensure your projects remain cutting-edge.