Dockerizing Your Flask Avatar API: A Step-by-Step Guide
Learn how to elevate your Flask app's reach by dockerizing and pushing it to Docker Hub, making deployment seamless and scalable.
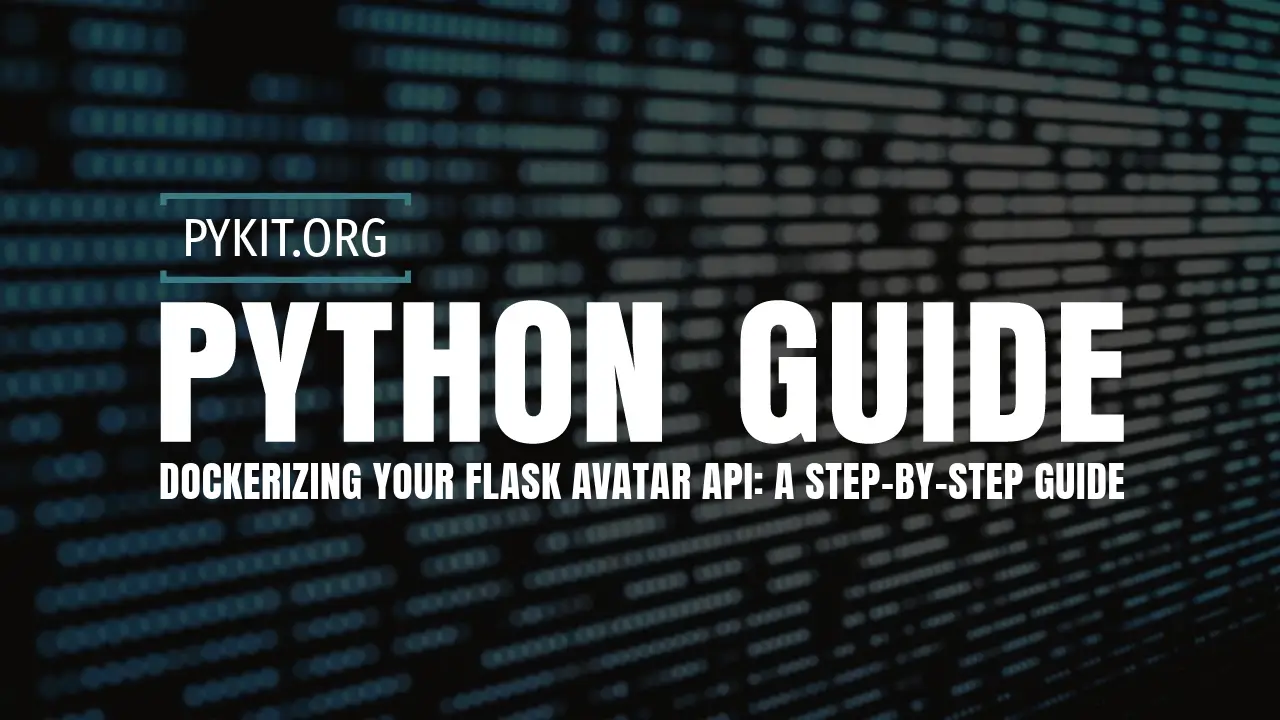
Dockerizing a Flask application not only streamlines the deployment process but also ensures consistency across development, testing, and production environments. This tutorial provides a detailed walkthrough of containerizing your Flask Avatar Generation API using Docker, ensuring it's ready for deployment with minimal hassle.
What You'll Need
- A functioning Flask Avatar API project.
- Docker installed on your machine.
- Basic familiarity with Flask, Python, and Docker concepts.
Step 1: Prepare Your Flask Application
Before dockerizing, ensure your Flask application is organized and includes a requirements.txt
file for dependencies. Your project structure might look something like this:
/Flask-Avatar-API
|-- app.py
|-- requirements.txt
|-- /templates
|-- /static
Your requirements.txt
should list Flask and Pillow, the two primary dependencies for the Avatar API:
Flask==2.0.1
Pillow==8.3.1
Step 2: Crafting Your Dockerfile
The Dockerfile is a blueprint for building your Docker image. It specifies the environment, files, and commands needed to run your Flask application inside a Docker container.
- Create the Dockerfile: In the root of your Flask project, create a file named
Dockerfile
.
Define the Entry Command: Specify how to run your application. For simplicity, we'll use Flask's built-in server, though Gunicorn or another WSGI server is recommended for production.
CMD ["flask", "run", "--host=0.0.0.0"]
Expose the Port: Flask runs on port 5000 by default. Expose this port to the Docker network.
EXPOSE 5000
Install Dependencies: Use requirements.txt
to install your Flask application's Python dependencies.
RUN pip install --no-cache-dir -r requirements.txt
Copy Your Application: Transfer your Flask application's code into the container.
COPY . .
Set the Working Directory: This directory is where your application will reside inside the container.
WORKDIR /app
Specify the Base Image: Use an official Python image as the starting point. This example uses Python 3.9.
FROM python:3.9-slim
Step 3: Building the Docker Image
With your Dockerfile
ready, build your Docker image. Open a terminal, navigate to your project directory, and run:
docker build -t flask-avatar-api .
This command creates a Docker image named flask-avatar-api
based on the instructions in your Dockerfile
.
Step 4: Running Your Flask Application in Docker
To run your Flask application inside a Docker container, execute:
docker run -p 5000:5000 flask-avatar-api
This command starts a container from your flask-avatar-api
image, mapping port 5000 inside the container to port 5000 on your host machine.
Step 5: Verifying the Application
Open a web browser and navigate to http://localhost:5000/avatar/<initials>
, replacing <initials>
with any two letters. You should see the generated avatar, confirming that your Flask application is running successfully inside a Docker container.
Step 6: Pushing Your Docker Image to a Registry
After successfully dockerizing your Flask application and testing it locally, the next step is to share or deploy your Docker image. This involves pushing it to a Docker registry—a service that hosts and distributes Docker images. Docker Hub is the most commonly used registry, but there are others like GitHub Container Registry, Google Container Registry, and AWS Elastic Container Registry (ECR).
Creating a Repository on Docker Hub
Before pushing your image, you need a repository on Docker Hub:
- Sign Up/Login: If you haven't already, sign up for Docker Hub or log in to your existing account.
- Create a New Repository: Navigate to the 'Repositories' tab and click 'Create Repository'. Name your repository (e.g.,
flask-avatar-api
) and provide a brief description.
Tagging Your Docker Image
Before you can push your image to Docker Hub, you must tag it with the repository name on Docker Hub. The tag generally includes your username, the repository name, and a version tag.
Tag the Image: Replace yourusername
with your Docker Hub username, and image_id
with your image's ID.
docker tag image_id yourusername/flask-avatar-api:latest
Find Your Image ID: List your Docker images to find the ID of the flask-avatar-api
image.
docker images
This command tags your local image as latest
in your Docker Hub repository.
Pushing the Image to Docker Hub
After tagging, push the image to your Docker Hub repository:
Push the Image: Use the docker push
command with your image tag.
docker push yourusername/flask-avatar-api:latest
Login to Docker CLI: If you haven't logged in to Docker Hub from your command line, do so using:
docker login
This command uploads your Docker image to Docker Hub, making it accessible for deployment on any server or development machine with Docker installed.
Verifying the Image in Docker Hub
- After pushing the image, go to your Docker Hub account, navigate to your repository, and you should see your
flask-avatar-api
image listed.
You can now pull this image on any machine with Docker installed using:
docker pull yourusername/flask-avatar-api:latest
Automating Docker Image Builds and Pushes
For a more streamlined workflow, especially in a team or CI/CD pipeline, consider automating the build and push process using GitHub Actions or other CI/CD tools. This can automatically build your Docker image and push it to Docker Hub whenever you make changes to your Flask application, ensuring your Docker images are always up to date.
Additional Considerations
- Development vs. Production: For production, consider using a more robust server like Gunicorn instead of Flask's built-in server. Update your
CMD
in the Dockerfile accordingly. - Environment Variables: Manage configuration and secrets using environment variables with Docker's
-e
or--env-file
options. - Logging and Monitoring: Implement logging and monitoring for your containerized application to ensure its health and performance.
Conclusion
You've successfully dockerized your Flask Avatar Generation API, encapsulating it in a portable container that can be easily deployed across different environments. Docker not only facilitates consistent development workflows but also significantly simplifies deployment and scaling processes. As you move forward, explore further optimizations and best practices for Docker and Flask to enhance your application's efficiency and resilience.
Pushing your Dockerized Flask application to Docker Hub is a crucial step in the container lifecycle, enabling you to share your application with others or deploy it across various environments easily. By following these steps, you've made your Flask application portable and ready for scalable deployment, further leveraging Docker's power for development and production use.