Building a Python Script to Backup Files Automatically: A Step-by-Step Guide
Learn how to create an automated backup script using Python. This guide covers everything from Python script for backup to automatic file backup Python techniques, ensuring your data is safely backed up.
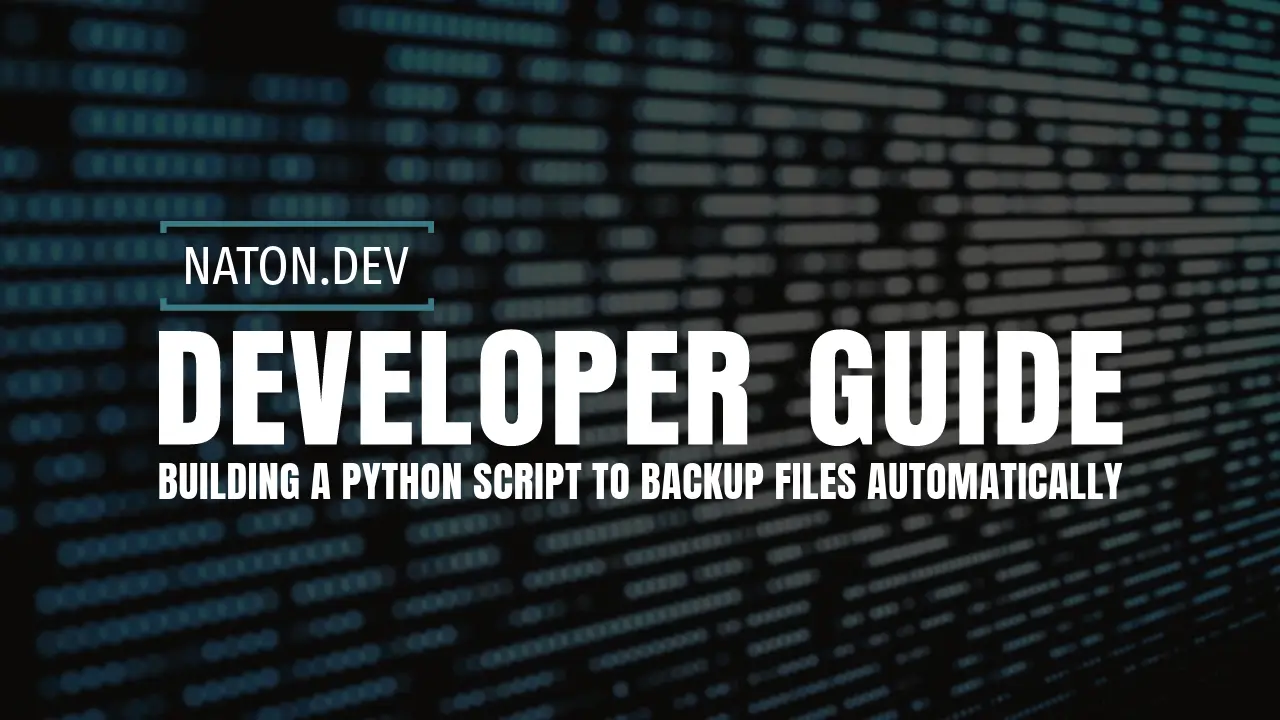
In the digital age, data loss is a significant risk that can affect anyone. This guide will show you how to safeguard your files by building a Python script for automatic backup, ensuring your precious data is always secure.
Understanding the Basics of Python Backup Automation
Automating backup processes using Python is not just efficient but also customizable to fit various backup needs. Whether you're looking to backup local files or ensure your cloud data is duplicated, Python offers robust libraries and tools to streamline these tasks. This section explores the fundamentals of Python backup automation, setting the stage for a deeper dive into creating your backup solution.
Setting Up Your Environment for Python Backup Files
Before diving into scripting, it's essential to prepare your environment. This involves installing Python and any necessary libraries. Setting up your Python environment for automated file backup is straightforward, and this guide will cover every step to ensure you're ready to script your backup process without a hitch.
Scripting Your Automatic File Backup Python Solution
Now, we'll get into the heart of the matter: scripting your automatic file backup Python solution. This part of the guide will provide detailed instructions on writing a Python script that automates the backup of your files. From selecting which files to backup to determining where these backups should be stored, we'll cover all the bases to ensure your script is both efficient and effective.
Automating Your Python Backup Files to Cloud
In an era where cloud storage has become ubiquitous, having the option to automate your Python backup files to cloud services like Google Drive and Dropbox is invaluable. This section will show you how to modify your script to upload backups directly to the cloud, providing an extra layer of security and accessibility to your data.
Scheduling Your Python Backup Script for Automation
The final piece of the puzzle is ensuring your backups occur regularly without manual intervention. Scheduling your Python backup script for automation can be achieved using various task schedulers depending on your operating system. This guide will outline how to schedule your backups, ensuring your data is always up-to-date and secure.
Step-By-Step Guide
Setting Up Your Environment for Python Backup Files
Step 1: Install Python: Ensure Python is installed on your system. If not, download and install it from the official Python website.
Step 2: Set Up a Virtual Environment: Create a virtual environment to manage your project's dependencies separately. Use the terminal or command prompt to navigate to your project directory and run:
python -m venv venv
Step 3: Activate the Virtual Environment:
On macOS and Linux, run:
source venv/bin/activate
On Windows, run:
venv\Scripts\activate
Step 4: Install Required Packages: Install boto3
if you're considering backing up to AWS S3, or any other library required for your backup destination.
pip install boto3
Scripting Your Automatic File Backup Python Solution
Step 1: Import Necessary Libraries: Start your script by importing any necessary libraries. For a simple file system backup, you might only need shutil
for file operations.
import shutil
import os
Step 2: Define Source and Destination: Specify the paths for the source directory you wish to backup and the destination where the backup should be stored.
source_folder = '/path/to/source'
backup_folder = '/path/to/backup'
Step 3: Script the Backup Process: Use shutil.copytree()
to copy the entire directory tree to the backup location. Handle any errors like directory already exists.
try:
shutil.copytree(source_folder, backup_folder)
print("Backup successful.")
except Error as e:
print(f"Error: {e}")
Automating Your Python Backup Files to Cloud
Step 1: Choose Your Cloud Service: Decide whether you're using AWS S3, Google Drive, or Dropbox and install the respective Python library for interacting with the service.
Step 2: Authenticate: Set up authentication by obtaining API keys or tokens from your cloud service provider and using them in your script.
Step 3: Modify the Script for Cloud Upload: Adjust your script to upload files to the cloud instead of copying to a local destination. For example, using boto3
for AWS S3:
import boto3
s3 = boto3.client('s3', aws_access_key_id='YOUR_ACCESS_KEY', aws_secret_access_key='YOUR_SECRET_KEY')
s3.upload_file('file_name.txt', 'bucket_name', 'file_name_in_s3.txt')
Scheduling Your Python Backup Script for Automation
Step 1: Determine the Scheduling Tool: Use cron
on Linux/macOS or Task Scheduler on Windows to run your backup script at regular intervals.
Step 2: Schedule the Script:
- On Windows, create a basic task in Task Scheduler to run the script daily at your preferred time.
On Linux/macOS, edit your crontab with crontab -e
and add a line like this for daily backups at 2 AM:
0 2 * * * /path/to/your/python /path/to/your/script.py
Conclusion
Building an automated backup script in Python is a smart way to protect your data from accidental loss. With the basics of Python backup automation covered in this guide, you're now equipped to script your way to a more secure data management routine.
Additional Learning Resources
To further enhance your understanding and capabilities in automating backup processes with Python, consider exploring the following resources. Each has been selected not only for its quality of content but also for its relevance to our key topics: Python scripting, automated file backup, cloud storage integration, and scheduling backup tasks.
- Python.org Documentation
Dive deeper into the fundamentals of Python programming with the official Python Documentation. It's a comprehensive resource for beginners and experienced developers alike, covering everything from basic syntax to advanced features like asynchronous programming. - Automate the Boring Stuff with Python
Al Sweigart’s Automate the Boring Stuff with Python is an excellent introduction to Python automation. The book includes practical examples that teach how to automate tasks such as managing files, working with spreadsheets, and more, making it a perfect companion for those looking to streamline their backup processes. - Using Python for Cloud Data Management
For those interested in cloud backups, this Cloud Data Management With Python guide from Real Python offers insights into how Python can interact with cloud services like AWS, Google Cloud, and Azure. Learn how to authenticate, upload, and manage your backup files in the cloud, leveraging Python scripts for efficient data management. - Cron Jobs and Task Scheduling
Task scheduling is crucial for automating your backups. The Cron Jobs for Pythonistas guide by Full Stack Python provides a detailed look at scheduling tasks withcron
on Linux and macOS. Windows users can benefit from tutorials on using Windows Task Scheduler for running Python backup scripts at set intervals. - GitHub Repositories on Python Backup Scripts
Explore various GitHub repositories tagged withpython-backup-script
for real-world examples and community-driven projects. These repositories can offer insights into how others approach file backup automation, including code for cloud storage integration and scheduling.
By exploring these resources, you'll gain a deeper understanding of how to leverage Python for automating file backups and much more. Whether you're looking to refine your script's efficiency, extend its functionality to the cloud, or ensure your backups run like clockwork, these resources provide a wealth of knowledge to help you succeed.