Beginner's Guide to Web Scraping with Python
In this tutorial, we'll explore how to build a simple web scraper using Python.
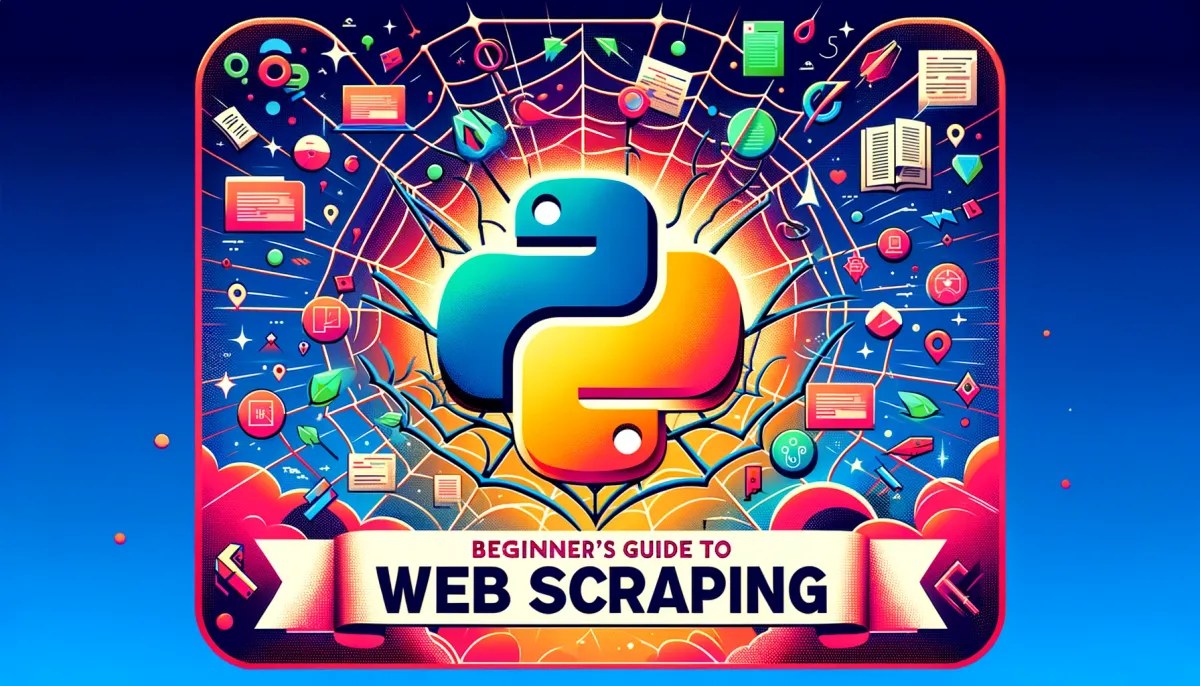
In this tutorial, we'll explore how to build a simple web scraper using Python. Web scraping is a powerful tool for automated data collection, allowing you to extract information from websites programmatically. We'll use Python 3 and two of its libraries: requests
for fetching web pages and BeautifulSoup
from bs4
for parsing HTML content. By the end of this tutorial, you'll know how to scrape data from a static webpage.
Prerequisites
- Basic understanding of Python.
- Python 3 installed on your machine.
- Familiarity with HTML and the structure of web pages.
Step 1: Install Required Libraries
First, ensure you have the necessary libraries installed. Open your terminal or command prompt and run:
pip install requests beautifulsoup4
Step 2: Fetch the Web Page
Choose a webpage you want to scrape. For this tutorial, we'll use a generic example.com
as our target. However, when choosing real websites, respect their robots.txt
file and terms of service.
import requests
url = 'http://example.com'
response = requests.get(url)
# Ensure the request was successful
if response.status_code == 200:
html_content = response.text
print("Page fetched successfully!")
else:
print("Failed to retrieve the webpage")
Step 3: Parse HTML Content
Now, let's parse the HTML content of the page using BeautifulSoup.
from bs4 import BeautifulSoup
soup = BeautifulSoup(html_content, 'html.parser')
Step 4: Extract Information
Let's say we want to extract all the headings (h1, h2, h3) from the webpage. Here's how you can do it:
headings = soup.find_all(['h1', 'h2', 'h3'])
for heading in headings:
print(heading.get_text())
This code snippet finds all elements that are either h1
, h2
, or h3
tags and prints their text content.
Step 5: Going Further
You can extract links, images, specific sections, or any data you need by adjusting your search criteria with BeautifulSoup. For example, to extract all links from the webpage, you could use:
links = soup.find_all('a')
for link in links:
print(link.get('href'))
This finds all <a>
tags and prints their href
attribute, which contains the URL they point to.
Conclusion
Congratulations! You've just built a basic web scraper with Python. Web scraping opens up a vast landscape for data collection and analysis. Remember, when scraping websites, always do so responsibly, respecting the website's rules and the legal constraints around web data extraction.
This tutorial provides a foundation, but there's much more to learn. Explore more advanced topics like handling JavaScript-rendered content with Selenium or Scrapy for more complex scraping projects.
Happy scraping!