Automating Docker Builds with GitHub Actions: A Step-by-Step Guide
Unlock continuous integration prowess by automating your Docker builds with GitHub Actions, streamlining your deployment process effortlessly.
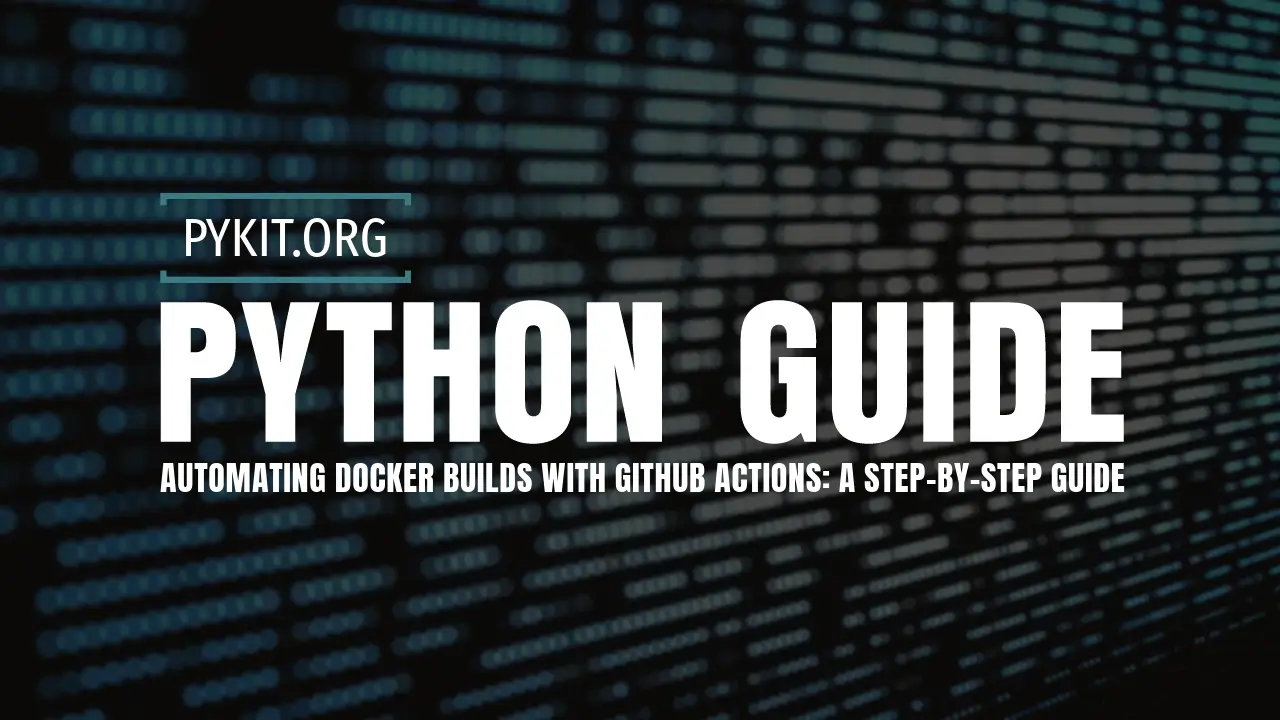
GitHub Actions offers a powerful automation tool that can build your Docker images and push them to Docker Hub whenever you make changes to your repository. This tutorial will guide you through setting up a workflow that automates the process of building a Docker image for your Flask Avatar API and pushing it to Docker Hub.
Prerequisites
- A GitHub account and a basic understanding of how to use Git.
- A Docker Hub account and a repository for your Docker image.
- A Flask application in a GitHub repository, ready to be dockerized.
Step 1: Preparing Your Repository
Ensure your repository contains all necessary files for your Flask application, including the Dockerfile
and any scripts or files required to run your application. Your repository should also include a .dockerignore
file to exclude files not needed in the Docker context, reducing the build time and size of your Docker image.
Dockerizing Your Flask Avatar API: A Step-by-Step Guide
Step 2: Setting Up Docker Hub Credentials as GitHub Secrets
To push images to Docker Hub, GitHub Actions will need your Docker Hub username and password (or access token). You should set these as secrets in your GitHub repository to keep them secure.
- Generate a Docker Hub Access Token: Log in to Docker Hub, navigate to
Account Settings
>Security
, and create a new access token. - Add Secrets to Your GitHub Repository: Go to your GitHub repository, click on
Settings
>Secrets
>New repository secret
, and add your Docker Hub username and access token as secrets. Name themDOCKER_USERNAME
andDOCKER_PASSWORD
, respectively.
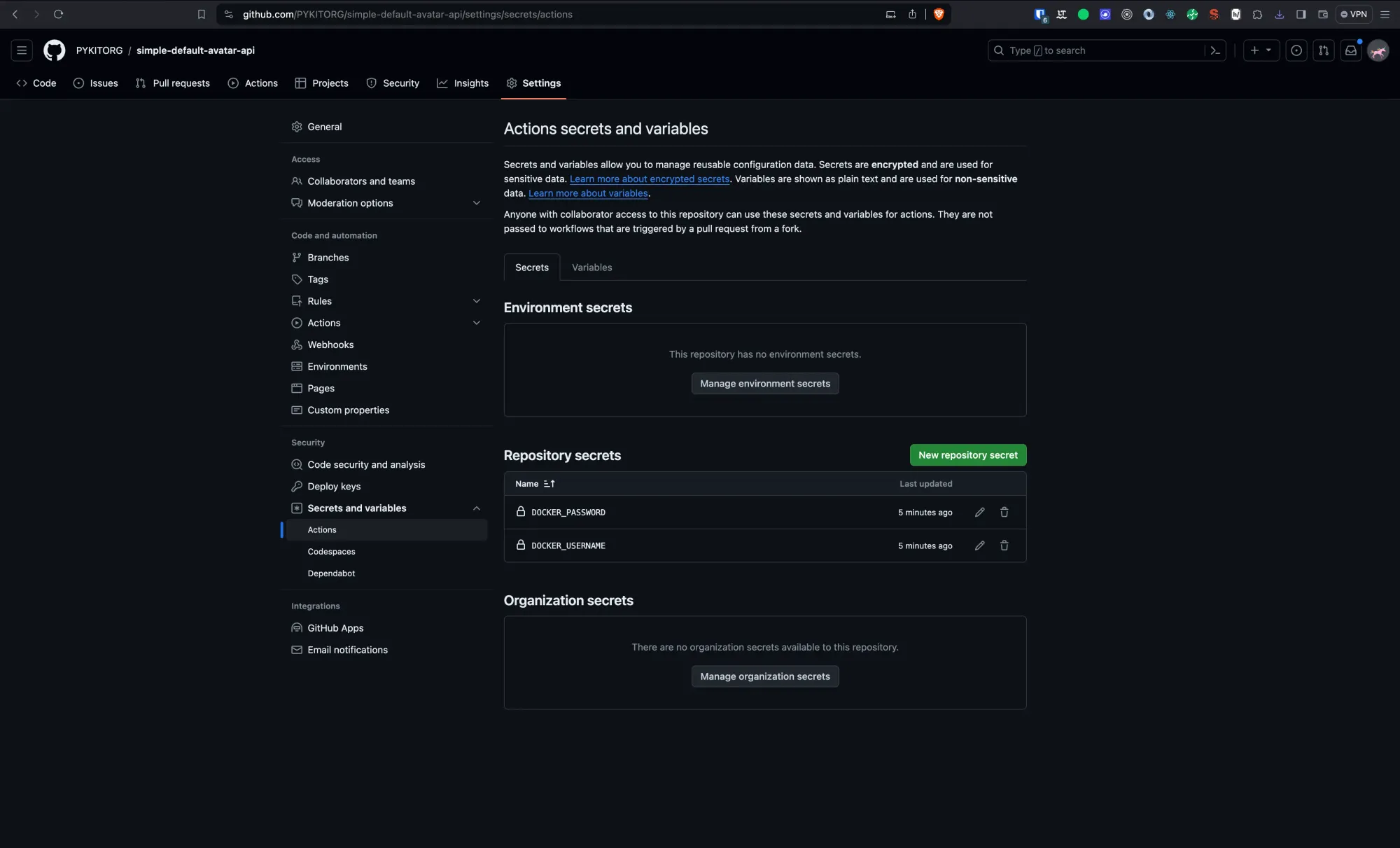
GitHub Secrets provide a secure way to store and manage sensitive information, such as passwords, access tokens, and private keys, within your GitHub repository. By using Secrets, you can avoid hard-coding sensitive data directly into your workflow files or source code, mitigating the risk of exposing confidential information.
When setting up automated Docker image builds and pushes with GitHub Actions, it's essential to use Secrets to handle your Docker Hub credentials safely. This approach allows GitHub Actions to authenticate with Docker Hub without revealing your username or access token in your workflow's logs or codebase.
Adding these credentials as Secrets—specifically naming them DOCKER_USERNAME
and DOCKER_PASSWORD
enables your GitHub Actions workflow to securely push newly built Docker images to your Docker Hub repository, ensuring a seamless and secure CI/CD pipeline.
Step 3: Creating the GitHub Actions Workflow
- Create a Workflow File: GitHub Actions are defined in special YAML files located in the
.github/workflows
directory at the root of your repository. If this directory doesn't exist, you'll need to create it. Inside, you create adocker-push.yml
file, which will hold the instructions for your CI/CD pipeline. - Defining the Workflow: The
docker-push.yml
file starts by specifying the name of the workflow and the conditions under which it should run. In this case, the workflow is triggered bypush
events to themain
branch. This setup ensures that every time changes are pushed tomain
, the workflow automatically kicks off.
name: Build and Push Docker image
on:
push:
branches:
- main
jobs:
build-and-push:
runs-on: ubuntu-latest
steps:
- name: Check Out Repository
uses: actions/checkout@v2
- name: Log in to Docker Hub
uses: docker/login-action@v1
with:
username: ${{ secrets.DOCKER_USERNAME }}
password: ${{ secrets.DOCKER_PASSWORD }}
- name: Build and Push Docker image
uses: docker/build-push-action@v2
with:
context: .
file: ./Dockerfile
push: true
tags: ${{ secrets.DOCKER_USERNAME }}/flask-avatar-api:latest
This workflow performs the following steps:
- Check Out Repository: The workflow begins with fetching the latest code from your repository using the
actions/checkout@v2
action. This step is necessary for the GitHub Actions runner to access the most current version of your codebase, including the Dockerfile required to build the Docker image. - Log in to Docker Hub: Before pushing the Docker image, the workflow must authenticate with Docker Hub to ensure you have the necessary permissions to upload images to your repository. This step uses the
docker/login-action@v1
action alongside your Docker Hub credentials stored as GitHub Secrets (DOCKER_USERNAME
andDOCKER_PASSWORD
). This secure approach keeps your credentials safe while allowing the workflow to log in on your behalf. - Build and Push Docker Image: The core of the workflow is the Docker image build and push operation, handled by
docker/build-push-action@v2
. This action performs several tasks:- It builds a Docker image from the Dockerfile located in your repository's root directory (
context: .
andfile: ./Dockerfile
). - It tags the built image with the specified tags. In this example, the image is tagged as
latest
, although you could use other strategies like semantic versioning or Git commit SHAs. - Finally, it pushes the tagged image to your Docker Hub repository, making it available for deployment.
- It builds a Docker image from the Dockerfile located in your repository's root directory (
Additional Considerations
- Tagging Strategy: The
latest
tag is convenient but might not be suitable for all use cases, especially in production environments where you need to track versions explicitly. Consider adopting semantic versioning or using GitHub Actions variables to tag images with the commit SHA for more control over versioning. - Security: Storing Docker Hub credentials as GitHub Secrets is a secure way to handle sensitive information. Ensure that access to these secrets is tightly controlled and regularly audited.
- Flexibility: This workflow is a basic example. GitHub Actions offers extensive customization options, including conditional execution, environment variables, matrix builds for testing across different environments, and more. Tailor the workflow to fit your project's specific CI/CD requirements.
By following these steps and leveraging GitHub Actions, you automate the tedious process of building and pushing Docker images, making your development workflow more efficient and error-proof.
Step 4: Triggering the Workflow
With your workflow defined, any push to the main branch of your repository will automatically trigger the GitHub Actions workflow. It will build your Docker image and push it to Docker Hub, streamlining your deployment process.
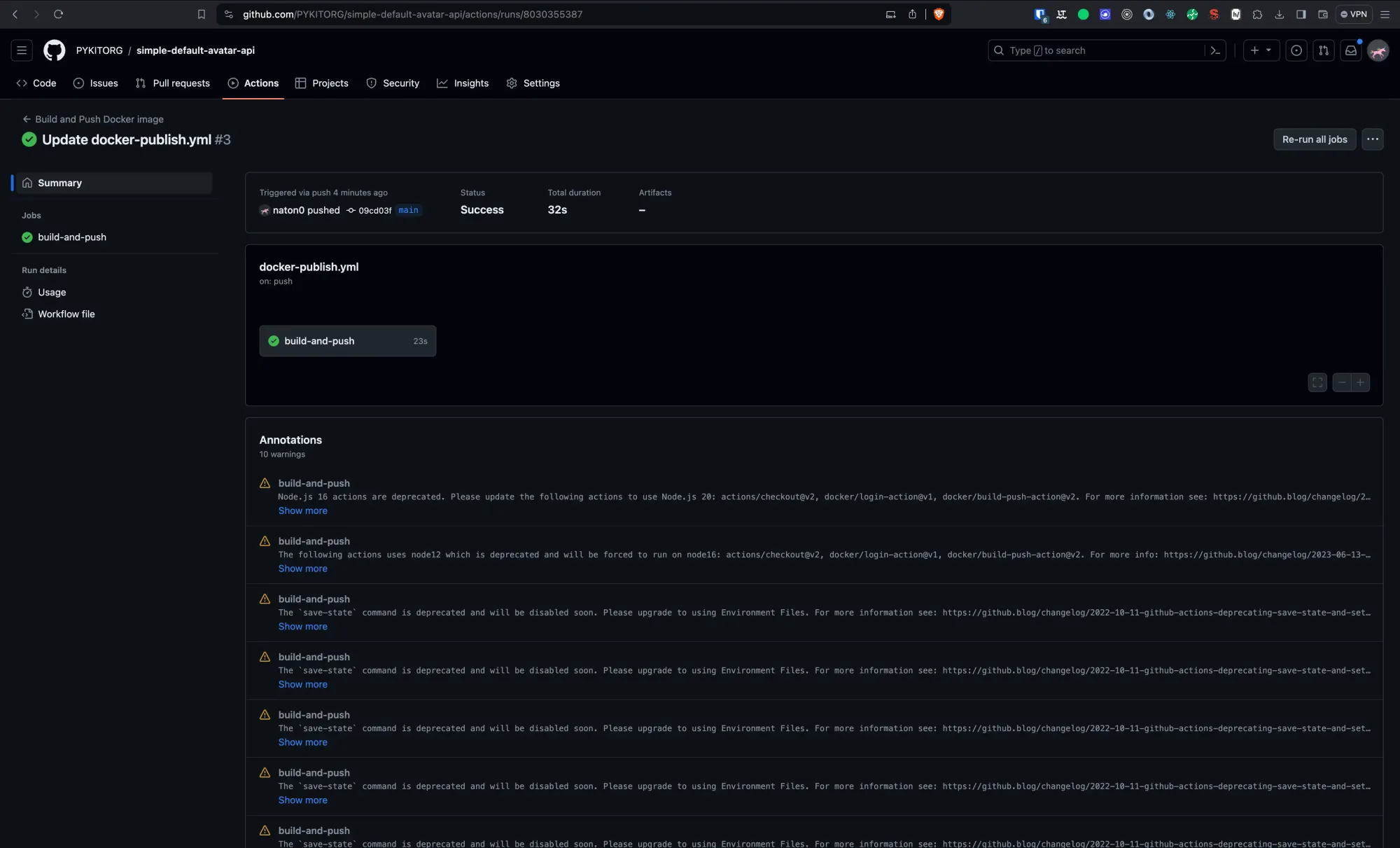
To enhance your GitHub Actions workflow for Docker image building and pushing by including the ability to manually retrigger or rerun jobs, you can add the workflow_dispatch
event to your existing configuration. This feature is particularly useful for scenarios where you might need to rebuild and push the Docker image without making any new commits to the main
branch, such as in the case of external dependencies changing or simply wanting to ensure the latest base image is used.
Step 5: Monitoring the Workflow
You can monitor the progress and outcome of your workflow runs directly from the GitHub repository under the Actions
tab. Here, you'll see a history of all workflow runs, including detailed logs that can help debug any issues that may arise.
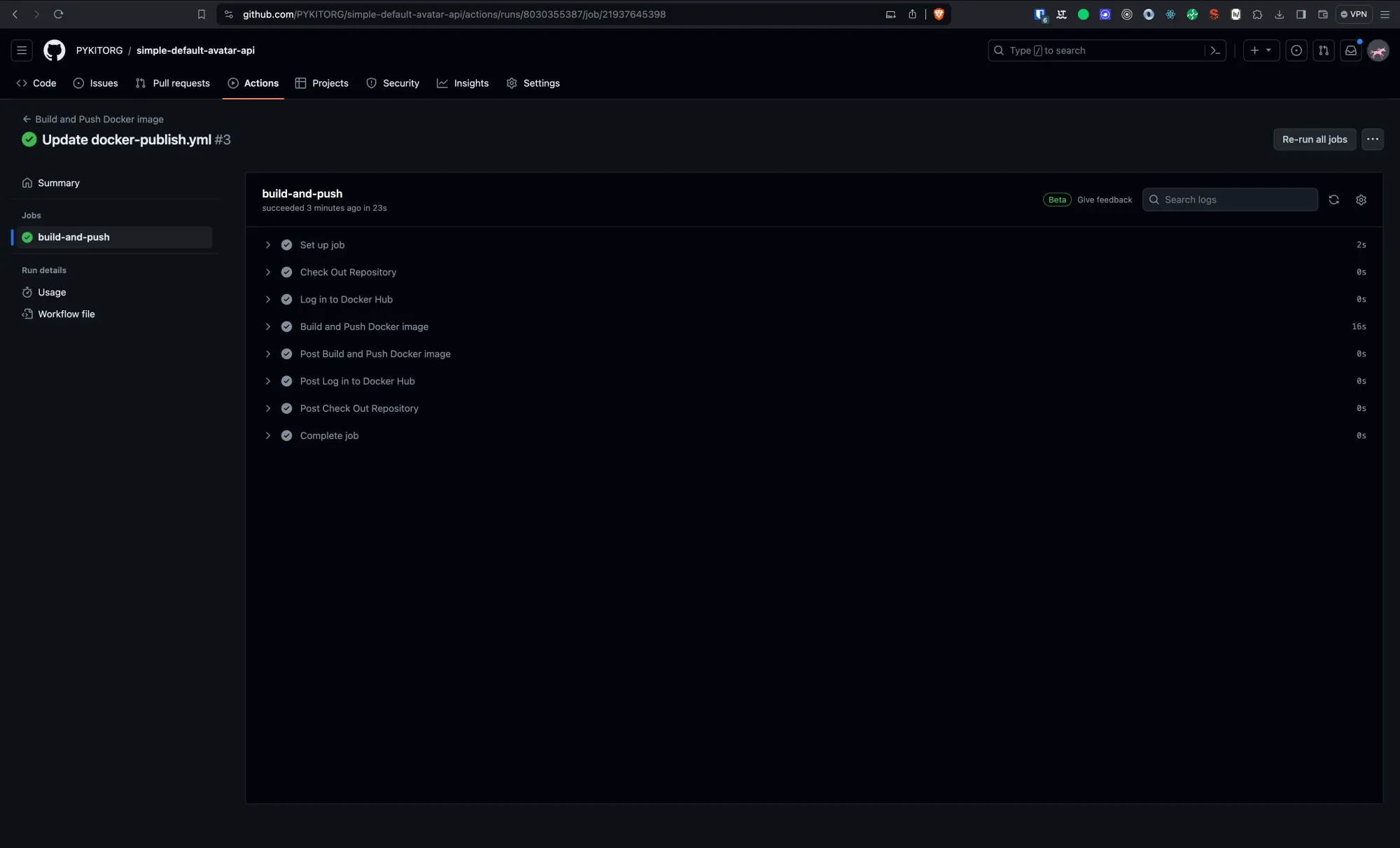
Conclusion
Automating Docker builds and pushes with GitHub Actions simplifies the deployment process and ensures that your Docker images are always up to date with your latest code. This tutorial provided a basic framework for setting up such automation, which can be customized and extended based on your project's specific needs and workflows.