A Beginner's Guide to Basic Python Commands
Dive into the world of programming with our beginner's guide to basic Python commands, unlocking the door to endless coding possibilities.
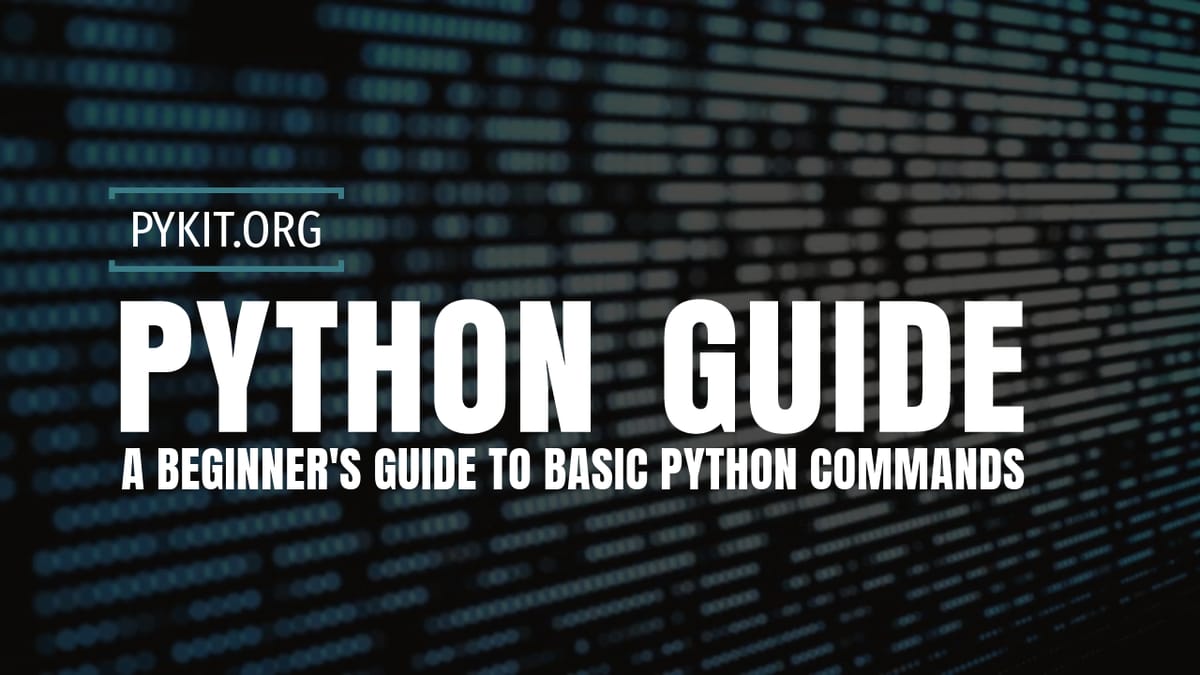
Python, known for its simplicity and readability, is a versatile programming language used across various domains, from web development to data science. For beginners embarking on their programming journey, mastering the basic commands is the first step towards building a solid foundation. This blog post introduces you to essential Python commands that every novice should know, providing a springboard into the world of programming.
Getting Started with Python
Before diving into the commands, ensure Python is installed on your computer. Python can be downloaded from the official website, and its installation is straightforward. Once installed, you can access the Python interpreter by typing python
or python3
(depending on your installation) in your terminal or command prompt.
1. Print Command
The print()
function is perhaps the most well-known command in Python. It outputs data to the standard output device (like your screen).
print("Hello, World!")
2. Comments
Comments are essential for making your code understandable. In Python, you use the #
symbol to start a comment.
# This is a single-line comment
3. Variables and Data Types
Variables are used to store information that can be referenced and manipulated. Python is dynamically-typed, meaning you don’t explicitly declare data types.
name = "John Doe" # String
age = 30 # Integer
height = 5.9 # Float
is_adult = True # Boolean
4. Arithmetic Operations
Python supports various arithmetic operators for performing mathematical calculations.
# Addition
print(5 + 3)
# Subtraction
print(10 - 2)
# Multiplication
print(4 * 2)
# Division
print(8 / 2)
# Modulus (remainder)
print(10 % 3)
# Exponentiation
print(2 ** 3)
5. Conditional Statements
Conditional statements allow you to execute certain lines of code based on specific conditions.
if age > 18:
print("You are an adult.")
else:
print("You are not an adult.")
6. Loops
Loops are used to iterate over a sequence (like a list, tuple, dictionary, set, or string) or execute a block of code multiple times.
# For loop
for i in range(5):
print(i)
# While loop
count = 0
while count < 5:
print(count)
count += 1
7. Functions
Functions are blocks of code designed to perform a specific task and can be reused.
def greet(name):
print(f"Hello, {name}!")
greet("Alice")
8. Lists and Dictionaries
Lists and dictionaries are data structures that store collections of items.
# List
fruits = ["apple", "banana", "cherry"]
print(fruits[0]) # Accessing the first item
# Dictionary
person = {"name": "John", "age": 30}
print(person["name"]) # Accessing the value of the key 'name'
Conclusion
These basic Python commands and concepts lay the groundwork for your programming journey. As you become more comfortable with these fundamentals, you'll find that Python's simplicity and power can help you create complex and efficient programs. Remember, practice is key to mastering any skill, so keep experimenting with these commands and explore more complex projects as you progress. Python is a language that grows with you, offering endless possibilities for development and innovation.